How Does The 4×4 Keypad Works And How To Use It With Arduino
How Does The 4×4 Keypad Works

The 4×4 keypad is composed of 16 buttons connected in a certain way as shown in the next figure. Each end of a wire (4 horizontal pink wires and 4 vertical violet wires) will represent a pin.
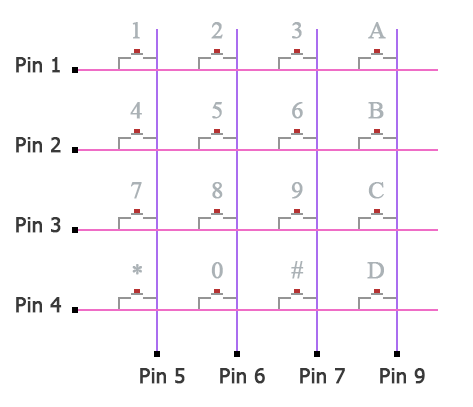
For example, when button number 5 is pressed, Pin 2 and Pin 6 are connected and to know that using the Arduino we have to follow the following instruction.
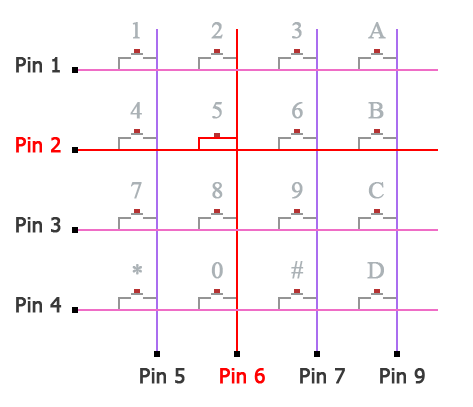
- Set pins 1, 2, 3, and 4 to a HIGH level and pins 5, 6, 7, and 8 to a LOW level.
- Check which Pin from pins 1, 2, 3, and 4 went to a LOW level. The pin will go to a LOW level because in the microcontroller here, we used the internal pull-up resistor to set it to a HIGH level, and when we ground the pin it will no longer stay pulled up HIGH and it will go LOW.
- Memorize pin 2 because it went LOW.
- Set each pin sequencly from pins 5, 6, 7, and 8 to a HIGH level and check if pin 2 went back to a HIGH level. when pin 2 went back to LOW check which pin from pins 5, 6, 7, and 8 led pin 2 to be HIGH (in this case pin 6).
- Check which symbol, number, or letter corresponds to pin 2 and pin 6.
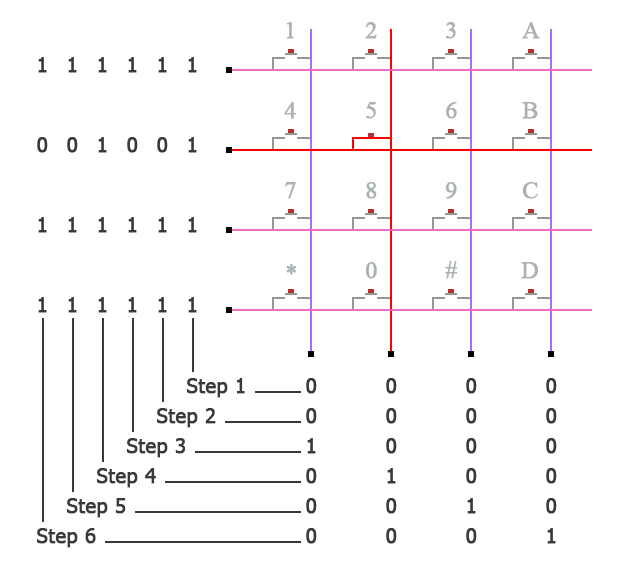
How To Use The 4x4 Keypad With Arduino
Unisng Mark Stanley And Alexander Brevig Library
In the Arduino IDE, navigate to Sketch > Include Library > Manage Libraries, then write in the search bar keypad, then install the one by Mark Stanley and Alexander Brevig.
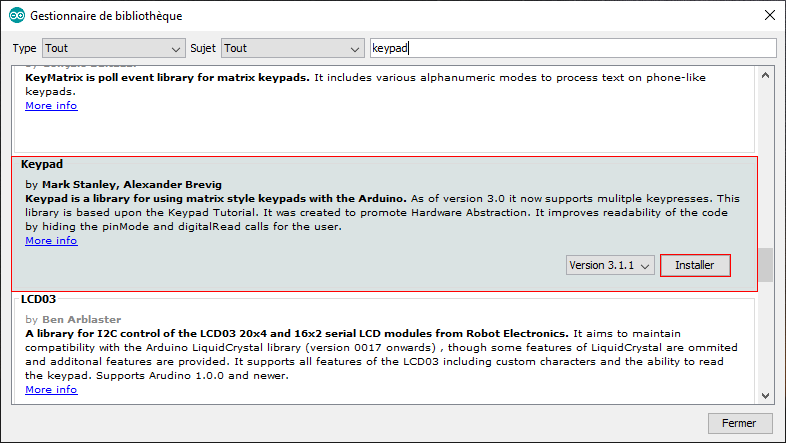
Schematic
Connect your keypad to the Arduino like it is shown in the next schematic. Open Arduino IDE and upload the code. In the end, you can see the results on the serial monitor once you press any button on the keypad.
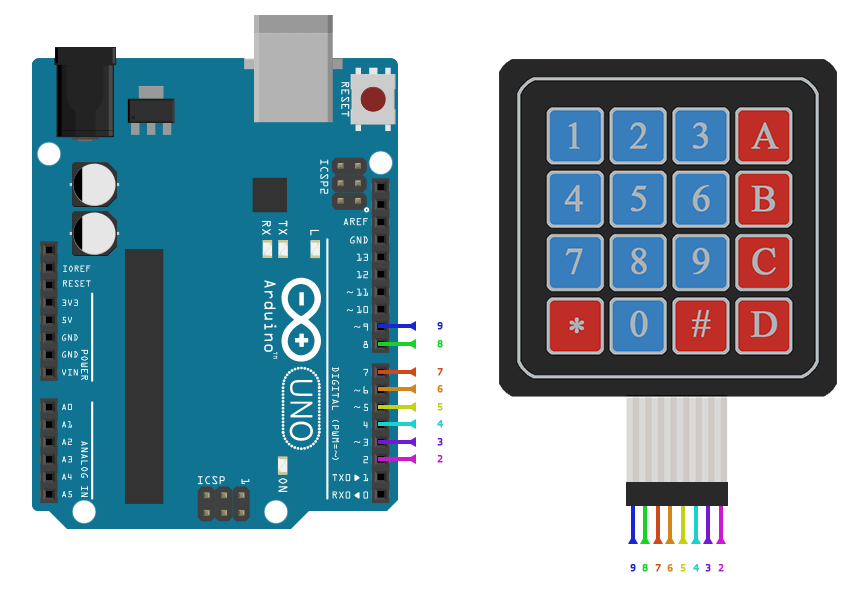
Arduino Code
#include<Keypad.h> const byte ROWS = 4; const byte COLS = 4; char keysTab[ROWS][COLS] = { {'1','2','3','A'}, {'4','5','6','B'}, {'7','8','9','C'}, {'*','0','#','D'} }; byte rowPins[ROWS] = {9, 8, 7, 6}; byte colPins[COLS] = {5, 4, 3, 2}; Keypad myKeypad = Keypad( makeKeymap(keysTab), rowPins, colPins, ROWS, COLS); void setup(){ Serial.begin(9600); } void loop(){ char Key = myKeypad.getKey(); if (Key){ Serial.println(Key); } }
Serial Monitor Results
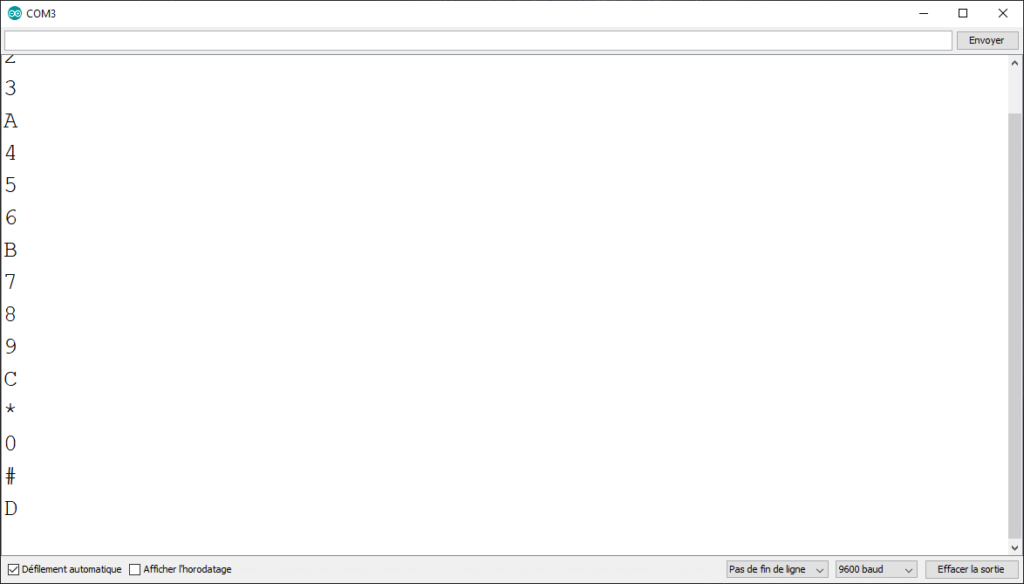
You may like to build!